What are data structures?
A data structure is a specialized format for organizing, processing, retrieving, and storing data.
Data structures are methods of storing and organizing data in a computer system so that operations can be performed more efficiently. When data is unstructured, it does not have a defined data model or is not organized in a manner
that is conducive to operations or analysis. Unstructured data is a common problem in organizations that have collected data but haven’t been storing or organizing it effectively. It is estimated that 80% of the world’s data is unstructured.
Data structures take the form of different layouts, each of which is efficient for some operations but inefficient for others. The goal of the program is to determine which data structures are suitable for the data on hand so that that data can be leveraged to solve problems.
Data structures provide a framework for representing and organizing record elements and their relationships, permitting diverse operations to be finished at the data in an extra prepared and optimized way.
Need for data structures
As programs have become extra complicated and the amount of statistics is increasing daily, which may cause problems with processing velocity, looking at records, managing more than one request, and so forth.
Data structure presents a manner of organizing, coping with, and storing facts correctly. With the assistance of statistics shape, the records items may be traversed easily.
Data shape offers efficiency, reusability, and abstraction. It plays an essential function in enhancing the performance of a program because the primary function of the program is to shop and retrieve the consumer’s statistics as speedily as viable.
The data types in the programming language are inadequate to apprehend the logical purpose for data processing and use.
A data structure provides meaning to the data and its data structure represents an Abstract Data Type (ADT) that confirms its relevance to the algorithm or application.
A data structure can include all types of data that are significant from the management, organization, and storage perspective. Thus, creating structured data ensures proper access to the respective.
A data structure helps in efficient data search and retrieval.
Problem-Solving: Many real-world problems involve complex relationships between data elements. Data structures provide the means to model these relationships effectively, making problem-solving and algorithm development more manageable.
Agile functionality: A well-designed data structure allows for faster execution of common tasks such as searching, sorting, and filtering. This speed is important when dealing with big data or time-sensitive applications.
Efficient Data Organization: Data structures allow for the organized storage and management of data. They enable efficient access, insertion, deletion, and modification of data elements, optimizing the performance of various operations.
Optimized Memory Usage: Different data structures offer varying levels of memory efficiency. By choosing the appropriate data structure for a specific task, you can reduce memory wastage and enhance the utilization of available resources.
Foundation for Software Development: Many programming languages and libraries offer built-in data structures as part of their standard libraries. Understanding these structures is essential for effective software development.
Support for Various Operations: Different data structures excel at different types of operations. For instance, hash tables are great for quick lookups, while trees are useful for maintaining hierarchical relationships.
Types of data structures
There are 2 types of Data Structures:
1. Primitive data structure types
2. Non-primitive data structure type.
Primitive data structure:
The data is stored one after the other in a consecutive manner. The data is arranged in a single layer. Irrespective of the volume of the data, these data structures are easy to use where the operations are simple. Each data stored is directly linked to its previous and next elements.
Uses: Linear data structures are widely used in software development.
Example: Array, Stack, Queue, Linked List, etc. Each of these data structures can be further subdivided into its types.
Non-linear Data structure:
The data is stored in a non-sequential (hierarchical) manner.
Layers of data: The data is arranged in multiple layers.
Time complexity: The time required to access all the data in the data structure remains the same even if the volume of the data increases.
Advantages: Irrespective of the volume of the data, these data structures are easy to use where the operations are complex.
Uses: Non-linear data structures are widely used in Artificial intelligence, image processing, etc.
Examples: Trees, Graphs, Tables, Sets, etc. Each of these data structures can be further subdivided into its types.
Data structures in java
Java is one of the most popular programming languages. It is high-level and object-oriented, and a Java program can be run infinitely on any platform that supports Java because it follows the “Write Once Run Anywhere” (WORA) principle.
Linear Data Structures
The following data structures are all linear, which means elements are sorted and searched sequentially, one after the other. There is one first element, one last element, and every element in between has a “next” and “previous.”
Arrays
The array represents one of the simplest types of data structure in Java. An array is a linear data structure, or “list,” where elements are stored.
It is continuous memory location data stored.
The array can also be sorted using techniques like quick sort or merge sort, but the biggest downside is that the size of the array is fixed.
All in all, you might use arrays if you need to store things linearly and especially if you need to search the elements frequently.
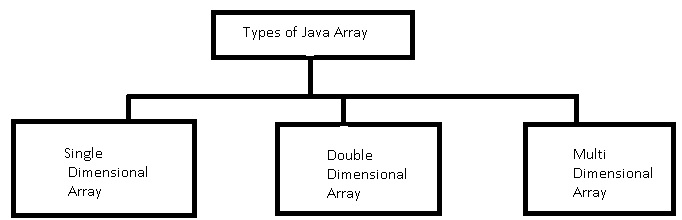
Stack
A Stack is a Last in First out (LIFO) data structure. It supports two basic operations called push and pop. The push operation adds an element at the top of the stack, and the pop operation removes an element from the top of the stack.
Java provides a Stack class that models the Stack data structure. The Stack class is part of Java’s collections framework.
The Stack class extends Vector which implements the List interface. A Vector is a re-sizable collection. It grows its size to accommodate new elements and shrinks the size when the elements are removed.
Queues
Queues follow the first in, first out (FIFO) method of organization. Using the queue, elements are added to the end of the list and deleted from the start of the list.
The Priority Queue and Linked List are the most common classes used for queues.
Queues are best utilized for storing elements before processing, and the FIFO principle ensures that they are processed in a given order.
Depending on your needs, you may use a circular queue for better memory utilization or a deque (double-ended queue) where insertions and deletions can take place from both ends.
Non-Linear Data Structures
The following data structures are all non-linear, which means there is no single sequential path connecting all the elements.
Instead, multiple paths may connect elements, allowing Java to efficiently accommodate a greater variety of applications and representing development complexity.
Binary Tree
The binary tree data structure in Java is not linear but rather, hierarchical. This means that the top-most element is the “root” of the tree, and all other nodes connect to it.
Each node in the tree can have up to two children. The best part about this data structure in Java is that you can access elements randomly.
The file system hierarchy of this structure allows for simple relationships among data to be easily represented. Plus, you can efficiently insert data and search the nodes with ease.
Graph
The graph data structure in Java is easy to imagine because it is quite literally a graph created by a grouping of edges and vertices.
The graph is represented using V(G) for the vertices and E(G) for the edges, with the “G” standing for the graph. Altogether, you end up with G(V, E) to summarize the graph.
Graphs are great because they can be disjointed or connected, directed or undirected, and so on. They’re flexible and powerful when it comes to finding connections and identifying the shortest path, which means they’re great for efficiency.
Hash
The hash data structure in Java uses the special hash function, which maps elements to a specific address where they are stored.
Variations include the hashmap and HashSet. The primary advantage of this data structure is that you get “constant-time” access. When collisions occur, two techniques chaining and open addressing used to resolve them.
When fetching data using the hash data structure in Java, you will need to use the Hash function. The great news is that hash is fantastic for helping you fetch data quickly, and it’s a highly efficient way to store elements.
Data structures in python
Data structures in Python refer to the various ways in which data can be organized, stored, and manipulated in the programming language.
Python provides built-in data structures such as lists, tuples, sets, and dictionaries. These data structures allow for efficient storage and retrieval of data, and they can be easily modified and manipulated as needed. Additionally, Python also supports more advanced data structures such as arrays, stacks, queues, and linked lists, which can be implemented using built-in classes or through third-party libraries.
Overall, data structures in Python provide the foundation for effective data management and programming in the language.
data structures are those that we can modify – for example, by adding, removing, or changing their elements.
Python has three mutable data structures: lists, dictionaries, and sets. Immutable data structures, on the other hand, are those that we cannot modify after their creation.
The only basic built-in immutable data structure in Python is a tuple.
Lists in Python
Lists are formed by enclosing elements within [square] brackets and separating each item with a comma.
#creating a list
test_list = ['Doctor', Engineer','Architect']
print(type(test_list))
print(test_list)
Output:
Doctor
Engineer
Architect
Appending values in Lists
We can add new members to an existing list using the append() or insert() methods.
append()
#appending the elements to the list
test_list = ['lawyer', 'Architect', 'Engineer', 'Doctor', 'Accountant']
test_list.append('Designer')
print(test_list)
OutPut: ['lawyer', 'Architect', 'Engineer', 'Doctor', 'Accountant', 'Designer']
INSERT()
#inserting the elements to the list
test_list = ['Accountant', 'Engineer', 'lawyer', 'Architect', 'Doctor']
test_list.insert(1, 'Designer')
print(test_list)
OutPut: ['Accountant', 'Designer', 'Engineer', 'lawyer', 'Architect', 'Doctor']
Removing Elements From Lists
pop() methods, we can remove elements from a list just like adding them using append(), insert(), or remove()
#removing the elements from the list
test_list = ['Engineer', 'Designer', 'lawyer', 'Architect', 'Doctor', 'Accountant']
test_list.remove('Designer')
print(test_list)
OutPut: ['Engineer', 'lawyer', 'Architect', 'Doctor', 'Accountant']
POP()
#removing the element at the particular index from the list
test_list = ['Designer', 'lawyer', 'Architect', 'Doctor', 'Accountant', 'Engineer']
test_list.pop(3)
print(test_list)
OutPut: ['Designer', 'lawyer', 'Architect', 'Accountant', 'Engineer']
Tuples in Python
Tuples are used to store multiple items in a single variable.
Tuple data type in Python is a collection of various immutable Python objects separated by commas. Tuples are similar to Python Lists, but they are syntactically different, i.e., in lists, we use square brackets while in tuples we use parentheses.
Example:
country = ('brazil', 'india', 'u.s.a')
print(type(country))
print(country)
OutPut: < class 'tuple' >
('brazil', 'india', 'u.s.a')
Set in Python
Sets are used to store multiple items in a single variable.
Sets are written with curly brackets.
Example:
ram = {“graphs”, “banana”, “cherry”}
print(ram)
Output: {‘graphs’, ‘banana’, ‘cherry’}
Tuples in Python
Tuples are used to store multiple items in a single variable.
A tuple is a collection that is ordered and unchangeable.
Tuples are written with round brackets.
Example:
demo = (“blackberry”, “banana”, “cherry”)
print(demo)
Output: (‘apple’, ‘banana’, ‘cherry’)
Applications of data structures
There are the following applications of data structures
Compiler Design:
Data structures such as symbol tables, abstract syntax trees, and stacks are used in compiler design to parse, analyze, and translate source code into machine code or intermediate representations.
Operating Systems:
Data structures like queues, linked lists, and scheduling algorithms are used in operating systems for process management, memory management, and file system operations.
Games and Simulations:
Data structures like graphs and heaps are used in game development and simulations to model game worlds, manage game states, and perform AI-based decisions.
Cryptography:
Data structures like hash tables and arrays are used in cryptographic algorithms to manage keys, perform encryption, and hash data for integrity verification.
Finance and Trading:
Data structures like priority queues and binary search trees are used in financial systems for managing real-time data, order execution, and market analysis.
Robotics and Motion Planning:
Data structures like graphs and motion planning algorithms are used in robotics for the pathfinding and motion control of robotic systems.
Robotics and Motion Planning:
Data structures like graphs and motion planning algorithms are used in robotics for the pathfinding and motion control of robotic systems.
FAQ
What are Data Structures?
A data structure is a mechanical or logical way that data is organized within a program. The organization of data is what determines how a program performs. There are many types of data structures, each with its own uses. When designing code, we need to pay particular attention to the way data is structured.
For example, B-trees are particularly well-suited for the implementation of databases, while compiler implementations usually use hash tables to look up identifiers.
What are the basic operations performed on various data structures?
The basic operations performed on data structures are as follows:
- Insertion – Adds a new data element in the data structure.
- Deletion – Removes a data element in a data structure.
- Searching – This involves searching for a specified data element in a data structure.
- Traversal – Processing all data elements present in it.
- Merging – Combines two similar data structures to form a new data structure of the same type.
- Sorting – Arranging data elements of a data structure in a specified order.
What is Stack and where it can be used?
Stack is a linear data structure in which the order LIFO(Last In First Out) or FILO(First In Last Out) for accessing elements. The basic operations of the stack are: Push, Pop, Peek
Explain what is a linked list.
A linked list is nothing but a sequence of nodes. With this sequence, each node is connected to the following node. It forms a chain of data storage.
List out the areas where the data structure is applied.
The data structure is a vital aspect of handling data. The following are specific areas where the data structure is applied:
- Numerical analysis
- Operating systems
- A.I.
- Database management
- Statistical analysis
What is infix, prefix, and postfix in data structure?
The way to write arithmetic expressions is known as notation. There are three types of notations used in an arithmetic expression, i.e., without changing the essence or output of the expression. These notations are:
- Prefix (Polish) Notation – In this, the operator is prefixed to operands, i.e. ahead of operands.
- Infix Notation – In this, operators are used in between operands.
- Postfix (Reverse-Polish) Notation – In this, the operator is postfixed to the operands, i.e., after the operands.
Explain the terminology LIFO
LIFO stands for Last In First Out.
This process describes how the data is accessed, stored, and then retrieved. So the latest data that is stored in the database can be extracted first.
What is a Binary Search Tree?
This is an interesting type of tree, i.e. binary search tree because it follows certain parameters.
The left subtree of a node contains only nodes with keys lesser than the node’s key.
The right subtree of a node contains only nodes with keys greater than the node’s key.
The left and right subtree each must also be a binary search tree.
What is the merge sort? How does it work?
Merge sort is a divide-and-conquer algorithm for sorting the data. It works by merging and sorting adjacent data to create bigger sorted lists, which are then merged recursively to form even bigger sorted lists until you have one single sorted list.
What are the advantages of a binary search over a linear search?
A binary search is more efficient than a linear search because we perform fewer comparisons. With linear search, we can only eliminate one element per comparison each time we fail to find the value we are looking for, but with the binary search, we eliminate half the set with each comparison.
Binary search runs in O(log n) time compared to linear search’s O(n) time. This means that the more of the elements present in the search array, the faster is binary search compared to a linear search.
Define what is a stack.
The stack is considered a data structure where the top layer element can be accessed. The data is stored in the stack and every time when data is stored, it pushes the data downwards which enables the users to access the latest data from the top layers.
What is FIFO?
FIFO in data terminology stands as “First in, First Out”.
This process defines or depicts how the data is stored inserted and accessed in a queue. Within this process, the data that is inserted at the beginning of the queue will only be extracted or accessed first.
List out all the advantages of a linked list.
The important aspect or advantage of a linked list is that it is the perfect data structure where the data can be modified very easily. Also, it doesn’t matter how many elements are available on the linked list.
Explain the main difference between a PUSH and a POP.
The two main activities, i.e. Pushing and Popping apply the way data is stored and retrieved in an entity. So if you check in detail, a Push is nothing but a process where data is added to the stack. On the contrary, a Pop is an activity where data is retrieved from the stack. When we discuss data retrieval we only consider the topmost available data.
What is an array?
While referring to an array the data is stored and utilized based on the index and this number actually co-relates to the element number in the data sequence. So thus making it flexible to access data in any order. Within programming language, an array is considered as a variable having a certain number of indexed elements.
List out all different sorting algorithms that are available and state which sorting algorithm is considered the fastest.
The list of all sorting algorithms is below:
- Quicksort
- Bubble sort
- Balloon sort
- Radix sort
- Merge sort
Out of the above sorting options, none of the sorting algorithms can be tagged as the fastest algorithm, because each of these sorting algorithms is defined for a specific purpose.
So based on the data structure and data sets available the sorting algorithms are used.
Why do we use Big O instead of Big Theta(θ)
Because, when analyzing performance, we are usually only interested in the worst-case scenario. Knowing the upper bound is therefore sufficient. When it performs better than expected for a given input.
Some Algorithms don’t have tight bounds at all. Moreover, tight bounds are often more difficult to compute.
What is Data abstraction?
Data abstraction refers to providing only essential information to the outside world and hiding their background details, i.e., to represent the needed information in the program without presenting the details.
Data abstraction is a programming (and design) technique that relies on the separation of interface and implementation.
For example, your program can make a call to the sort() function without knowing what algorithm the function actually uses to sort the given values.
In fact, the underlying implementation of the sorting functionality could change between releases of the library, and as long as the interface stays the same, your function call will still work.
What is the bucket sort algorithm?
Bucket sort, also known as bin sort, is a sorting algorithm that splits the contents of an array into many buckets. The buckets are then sorted one by one, either with a separate sorting algorithm or by applying the bucket sorting process recursively.
Algorithm:
Create an empty array of size n(n empty buckets).
Loop through the original array and put each array element in a “bucket”.
Sort each of the non-empty buckets using insertion sort.
Visit the buckets in order and put all elements back into the original array
Complexity: Worst case time complexity:Θ(n2)
Average case time complexity:Θ(n+k)
Best case time complexity:Θ(n+k)
Space complexity:Θ(n+k)
Explain the Boyer-Moore Algorithm.
The Boyer-Moore algorithm is a searching algorithm that looks for a string with length n and a pattern with length m. It prints all of the pattern’s occurrences in the Text. Boyer Moore employs both a bad character heuristic and a positive character heuristic.
Each of them is utilized to look for the pattern on its own. Pattern processing is utilized to create separate arrays for both heuristics in this approach, and the best heuristic is used at each stage. Boyer Moore begins by matching the previous pattern, which differs from KMP and Naive’s method.
What is the difference between a binary tree and a binary search tree (BST)?
- Binary Tree: A binary tree is a hierarchical data structure in which each node has, at most, two child nodes, which are referred to as the left child and the right child. Binary trees are used for a wide range of applications, including expression evaluation and hierarchical data representation.
- Binary Search Tree (BST): A binary search tree is a binary tree with the additional property that for each node, all values in its left subtree are less than or equal to its value, and all values in its right subtree are greater than its value. BSTs are used for efficient searching, insertion, and deletion operations, with average time complexities of O(log n) for these operations in balanced trees.
Describe the difference between a stack and a queue.
- Stack: A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. It means that the last element added to the stack will be the first one to be removed. Stacks are typically used for tasks that involve maintaining a history, such as function call stacks in programming.
- Queue: A queue is also a linear data structure but follows the First-In-First-Out (FIFO) principle. In a queue, the first element added is the first one to be removed. Queues are commonly used in scenarios where tasks need to be processed in the order they arrive, such as job scheduling and breadth-first search algorithms.
What is a hash table, and what is its primary use?
A hash table is a data structure that stores data in an associative array format, where data is stored as key-value pairs. It uses a hash function to map keys to specific locations (buckets) in an array, making it efficient to retrieve values by their keys.
Hash tables are primarily used for fast data retrieval, making them suitable for implementing dictionaries, caches, and database indexing.
What is the difference between a list and a tuple in Python?
List | Tuple |
---|---|
Lists are mutable. They can be modified after the creation | Tuples are immutable. Once a tuple is created it cannot be changed Lists have ordered. |
Lists consume more memory | Tuple consumes less memory as compared to the list |
The list is better for performing operations, such as insertion and deletion. | A Tuple data type is appropriate for accessing the elements |
What is the time complexity of searching for an element in a sorted array using binary search?
The time complexity of binary search is O(log n), where ‘n’ is the number of elements in the array.
How does a bubble sort algorithm work, and what is its time complexity?
Bubble sort repeatedly swaps adjacent elements if they are in the wrong order. Its time complexity is O(n^2) in the worst case.
How does a depth-first search (DFS) algorithm work for traversing graphs?
DFS explores as far as possible along one branch before backtracking. It uses a stack (or recursion) for traversal.
What are the differences between a min-heap and a max-heap?
In a min-heap, the parent node is smaller than its children, and the root contains the smallest element. In a max-heap, the parent node is larger than its children, and the root contains the largest element.
How do you reverse a linked list?
You can reverse a linked list iteratively by changing the direction of the next pointers, or recursively by swapping and reversing pointers.
What is the time complexity of various operations in a hash table with good hashing and no collisions?
- Insertion: O(1)
- Deletion: O(1)
- Search: O(1)
How does an AVL tree maintain its balance, and why is balance important?
AVL trees maintain balance by ensuring that the height difference between the left and right subtrees of any node is at most one. Balance is crucial to guarantee efficient O(log n) insertions and deletions.
Explain the concept of a circular buffer, and when is it useful.
A circular buffer is a fixed-size buffer that wraps around, allowing efficient use of limited memory. It’s useful in scenarios like streaming data processing.
What is the time complexity of sorting algorithms like bubble sort, selection sort, and insertion sort?
Bubble sort, selection sort, and insertion sort all have a time complexity of O(n^2) in the worst case.
What is the time complexity of finding the shortest path in a weighted graph using Dijkstra’s algorithm?
The time complexity of Dijkstra’s algorithm is O(V^2) with a simple implementation using an adjacency matrix. With a priority queue, it’s O(E + V log V), where ‘E’ is the number of edges and ‘V’ is the number of vertices.
Explain the concept of a disjoint-set data structure (Union-Find) and its applications.
A disjoint-set data structure keeps track of a collection of disjoint sets, often represented as trees. It’s used for tasks like connected components in graphs and Kruskal’s algorithm for finding minimum spanning trees.
What is the difference between an array and a linked list?
Array | Linked List |
An array is a sequence of elements of a similar data type. | A Linked list is a set of objects known as a node, where it internally consists of two parts, i.e., data and address. |
It can be accessed irregularly using the array index. | Linked lists support random access. Only supports sequential access. |
Array elements are stored in contiguous locations in memory. | New elements can be stored anywhere, and a reference is created for the new element using pointers. |
In arrays, memory allocation is done during compile time. | In linked lists, memory allocation is done during runtime. |
Array size must be defined at the time of declaration/initialization. | The linked list size grows when new elements are inserted or deleted. |